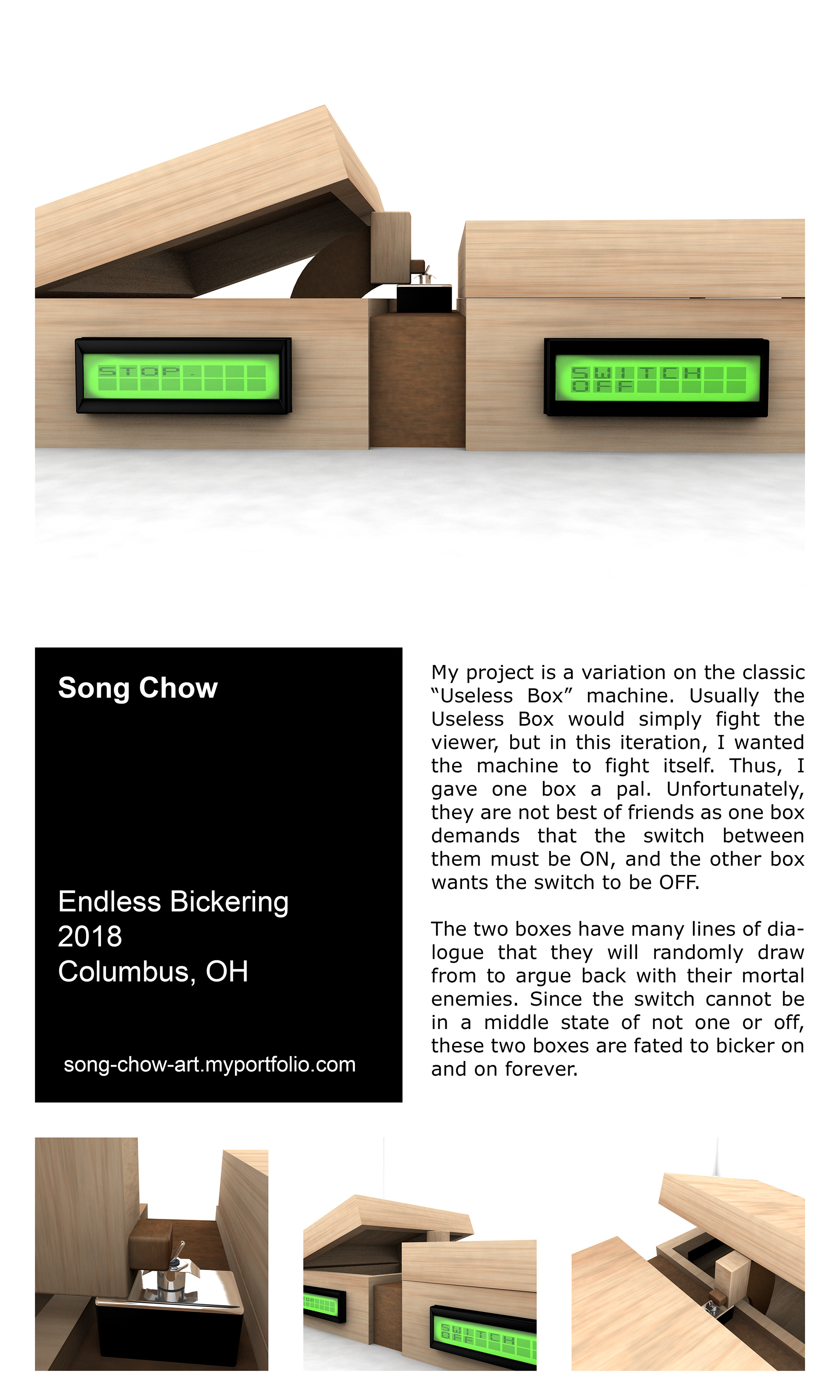
Regarding functionality and parts, my argue boxes now are able to react to a switch being turned on and off. When on, they will simply say to keep the switch off/on, while when turned off, the LCD screen will display a random angry phrase!
When the switch is turned on or off (depending on the box), the servo motor controlling the arm will now move towards and then back to its original position.
My biggest problem right now is that I am still trying to figuring out how to connect one switch to TWO Arduinos. I am going to try a DPDT switch.
Either way things are getting pretty close!
I have my protoboards ready to solder as well!
As of today, my project is pretty close to being done. The code is 90% done and the LCD screen is running as seen above.
Of course, the LCD screen now displays text depending on what the switch is doing, but I still haven't completely figured out how to have two arduinos talk to each other with one switch between them.
I also have soldered on some wires to the switch for ease of access.
And here is me, applying a delicate touch to the wiring. Right now most of the work comes down to making sure all the mechanisms work with each other.
Hopefully I can finish this piece peacefully and on time! We'll see!
Here is my Arduino code so far. It's pretty much done. For the other box, it's simply reversed with different argument lines.
#include <Servo.h>
#include <LiquidCrystal.h>
#include <LiquidCrystal.h>
int buttonPin = 8;//switch pin number
int buttonState;//button state is either HIGH or LOW
int highRandom;//random texts for high
int highRandom;//random texts for high
const int rs = 12, en = 11, d4 = 5, d5 = 4, d6 = 3, d7 = 2;//numbers of the pins of the lcd
LiquidCrystal lcd(rs, en, d4, d5, d6, d7);//I have no idea what this is
Servo myservo; // create servo object to control a servo
int pos = 0; //position of servo! change numbers to change position
void setup() {
lcd.begin(16, 2);//setup how many columns and rows are in the lcd
lcd.cursor();
lcd.begin(16, 2);//setup how many columns and rows are in the lcd
lcd.cursor();
pinMode(buttonPin, INPUT);//setup the button pin; button is an input, output is the motion
myservo.attach(9); // attaches the servo on pin 9 to the servo object
}
}
void loop() {
// if they're different
if (buttonState != digitalRead(buttonPin)) {
buttonState = digitalRead(buttonPin);//check the button
if (buttonState == HIGH) {
highRandom = int(random(1, 13));//random does not include the max number
if (highRandom == 1) {
SayStuff("Stop it.",0,0);
}
if (highRandom == 2) {
SayStuff("Seriously stop.",0,0);
}
if (highRandom == 3) {
SayStuff("This is annoying.",0,0);
}
if (highRandom == 4) {
SayStuff("Can you not?",0,0);
}
if (highRandom == 5) {
SayStuff("STOP.",0,0);
}
if (highRandom == 6) {
SayStuff("I'm so upset.",0,0);
}
if (highRandom == 7) {
SayStuff("This is rude.",0,0);
}
if (highRandom == 8) {
lcd.clear();
lcd.setCursor(0,0);
lcd.print("Who made you");
lcd.setCursor(0,1);
lcd.print("this way?");
}
if (highRandom == 9) {
SayStuff("S T O P",0,0);
}
if (highRandom == 10) {
lcd.clear();
lcd.setCursor(0,0);
lcd.print("Why are you");
lcd.setCursor(0,1);
lcd.print("this way?");
}
if (highRandom == 11) {
SayStuff("For real?",0,0);
}
if (highRandom == 12) {
SayStuff("I hate you.",0,0);
}
for (pos = 0; pos <= 70; pos += 1) { // goes from 0 degrees to 180 degrees
// in steps of 1 degree
myservo.write(pos);
delay(15); // tell servo to go to position in variable 'pos'
}
for (pos = 70; pos >= 0; pos -= 1) { // goes from 180 degrees to 0 degrees
myservo.write(pos);
delay(15); // tell servo to go to position in variable 'pos'
}
}
else if (buttonState == LOW) {
lcd.clear();
lcd.setCursor(0,0);
lcd.print("Please keep");
lcd.setCursor(0,1);
lcd.print("switch off.");
}
}
}
// if they're different
if (buttonState != digitalRead(buttonPin)) {
buttonState = digitalRead(buttonPin);//check the button
if (buttonState == HIGH) {
highRandom = int(random(1, 13));//random does not include the max number
if (highRandom == 1) {
SayStuff("Stop it.",0,0);
}
if (highRandom == 2) {
SayStuff("Seriously stop.",0,0);
}
if (highRandom == 3) {
SayStuff("This is annoying.",0,0);
}
if (highRandom == 4) {
SayStuff("Can you not?",0,0);
}
if (highRandom == 5) {
SayStuff("STOP.",0,0);
}
if (highRandom == 6) {
SayStuff("I'm so upset.",0,0);
}
if (highRandom == 7) {
SayStuff("This is rude.",0,0);
}
if (highRandom == 8) {
lcd.clear();
lcd.setCursor(0,0);
lcd.print("Who made you");
lcd.setCursor(0,1);
lcd.print("this way?");
}
if (highRandom == 9) {
SayStuff("S T O P",0,0);
}
if (highRandom == 10) {
lcd.clear();
lcd.setCursor(0,0);
lcd.print("Why are you");
lcd.setCursor(0,1);
lcd.print("this way?");
}
if (highRandom == 11) {
SayStuff("For real?",0,0);
}
if (highRandom == 12) {
SayStuff("I hate you.",0,0);
}
for (pos = 0; pos <= 70; pos += 1) { // goes from 0 degrees to 180 degrees
// in steps of 1 degree
myservo.write(pos);
delay(15); // tell servo to go to position in variable 'pos'
}
for (pos = 70; pos >= 0; pos -= 1) { // goes from 180 degrees to 0 degrees
myservo.write(pos);
delay(15); // tell servo to go to position in variable 'pos'
}
}
else if (buttonState == LOW) {
lcd.clear();
lcd.setCursor(0,0);
lcd.print("Please keep");
lcd.setCursor(0,1);
lcd.print("switch off.");
}
}
}
void SayStuff(String stuff, int row, int column) { //say stuff function
//clears screen
lcd.clear();
// reset the cursor. Curos is ROW, COL
lcd.setCursor(row, column);
// write to screen
lcd.print(stuff);
}
//clears screen
lcd.clear();
// reset the cursor. Curos is ROW, COL
lcd.setCursor(row, column);
// write to screen
lcd.print(stuff);
}